建造者模式
模式定义:将一个复杂对象的创建与它的表示分离,使得同样的构建过程创建不同的表示
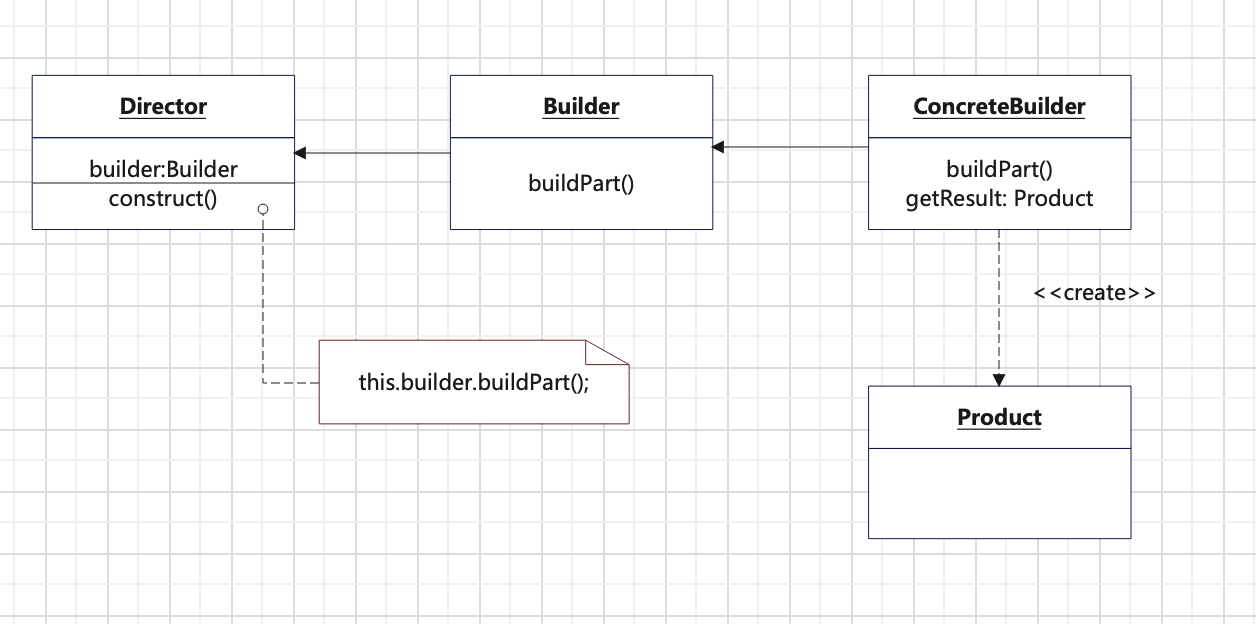
忽视Director、Product类,只关注建造本身
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
| public class ComputerBuilder{ private String cpu; private String motherboard; private String monitor; private String keyboard; private String mouse;
public ComputerBuilder(String cpu, String motherboard, String monitor, String keyboard, String mouse) { this.cpu = cpu; this.motherboard = motherboard; this.monitor = monitor; this.keyboard = keyboard; this.mouse = mouse; }
@Override public String toString() { return "ComputerBuilder{" + "cpu='" + cpu + '\'' + ", motherboard='" + motherboard + '\'' + ", monitor='" + monitor + '\'' + ", keyboard='" + keyboard + '\'' + ", mouse='" + mouse + '\'' + '}'; }
public static Builder newBuilder(){ return new Builder(); }
public static class Builder{ private String cpu; private String motherboard; private String monitor; private String keyboard; private String mouse;
public Builder() { }
public Builder setCpu(String cpu) { this.cpu = cpu; return this; }
public Builder setMotherboard(String motherboard) { this.motherboard = motherboard; return this; }
public Builder setMonitor(String monitor) { this.monitor = monitor; return this; }
public Builder setKeyboard(String keyboard) { this.keyboard = keyboard; return this; }
public Builder setMouse(String mouse) { this.mouse = mouse; return this; }
public ComputerBuilder builder(){
return new ComputerBuilder(cpu,motherboard,monitor,keyboard,mouse); } } }
|
普通链式调用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97
| class Product{ private String name;
private int count;
private Double weight;
public Product(String name, int count, Double weight) { this.name = name; this.count = count; this.weight = weight; }
public Product() {
}
public String getName() { return name; }
public int getCount() { return count; }
public Double getWeight() { return weight; }
public void setName(String name) { this.name = name; }
public void setCount(int count) { this.count = count; }
public void setWeight(Double weight) { this.weight = weight; }
@Override public String toString() { return "Product{" + "name='" + name + '\'' + ", count=" + count + ", weight=" + weight + '}'; } }
interface ProductBuilder{ ProductBuilder makeName(String name); ProductBuilder makeCount(int count); ProductBuilder makeWeight(Double weight);
public Product getProduct(); }
class ConcreteProductBuilder implements ProductBuilder{
private Product product = new Product();
@Override public ProductBuilder makeName(String name) { product.setName("can"); return this; }
@Override public ProductBuilder makeCount(int count) { product.setCount(10); return this; }
@Override public ProductBuilder makeWeight(Double weight) { product.setWeight(20.0); return this; }
@Override public Product getProduct() { return product; } } class ProductDirector{
public void makeProduct(ProductBuilder builder){ builder.makeName("can"); builder.makeCount(1); builder.makeWeight(2.0); }
}
|
应用场景:
1.需要生产的对象具有复杂的内部结构
2.需要生成的对象内部属性本身相互依赖
3.与不可变对象配合使用
优点:
1、建造者独立,亦拓展
2、便于控制细节风险
Spring源码中的应用
1 2
| org.springframework.web.servlet.mvc.method.RequestMappingInfo; org.springframework.beans.factory.support.BeanDefinitionBuilder;
|