适配器模式
模式定义:
将一个类的接口转换成客户希望的另一个接口。Adapter模式使得原本由于接口不兼容而不能一起工作的那些类可以一起工作。
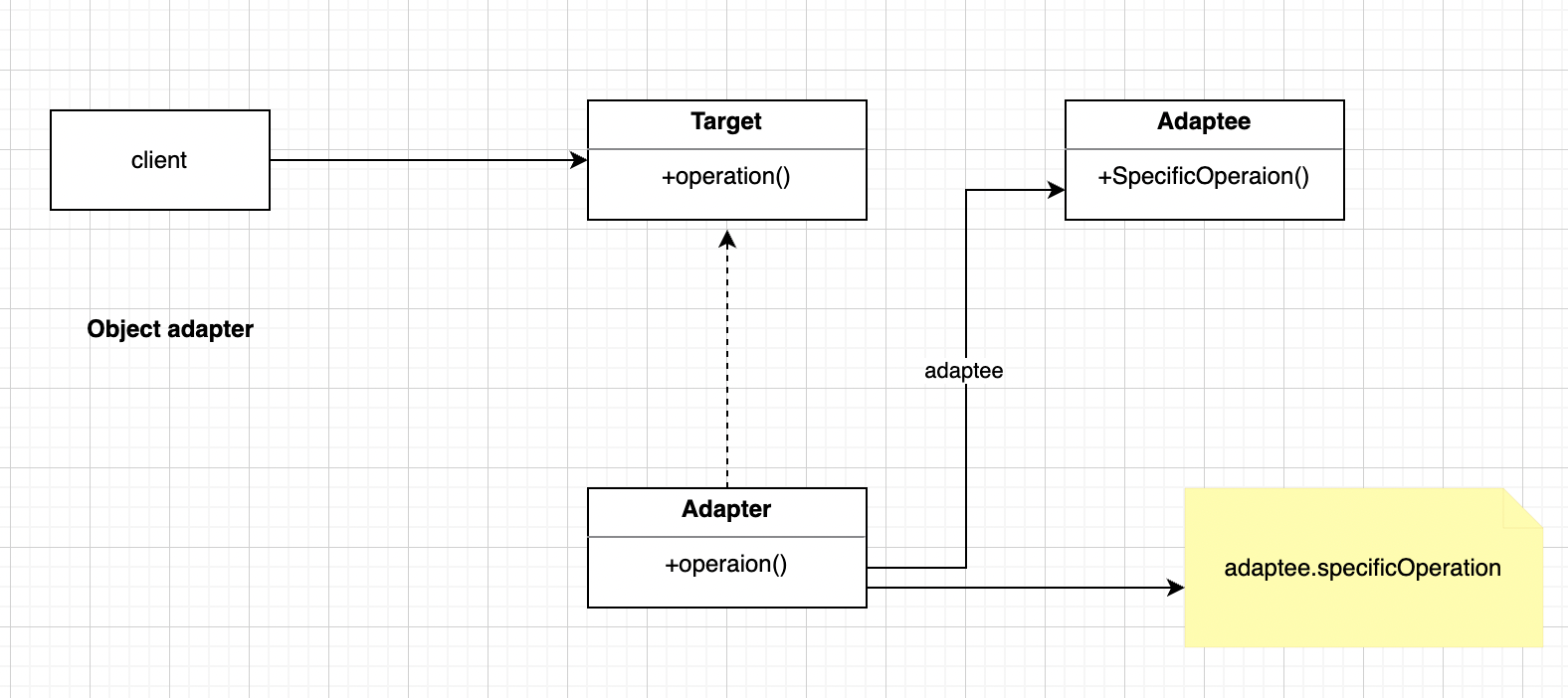
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public class ObjectAdapter { public static void main(String[] args) { Adaptee adaptee = new Adaptee(); Adapter adapter = new Adapter(adaptee); adapter.output5v(); } } class Adaptee{ public int outPut220v(){ return 220; } } interface Target{ int output5v(); } class Adapter implements Target {
private Adaptee adaptee;
public Adapter (Adaptee adaptee){ this.adaptee = adaptee; } @Override public int output5v() { int i = adaptee.outPut220v(); System.out.println(String.format("原始电压:%d v -> 输出电压: %d v",i,5)); return 5; } }
|
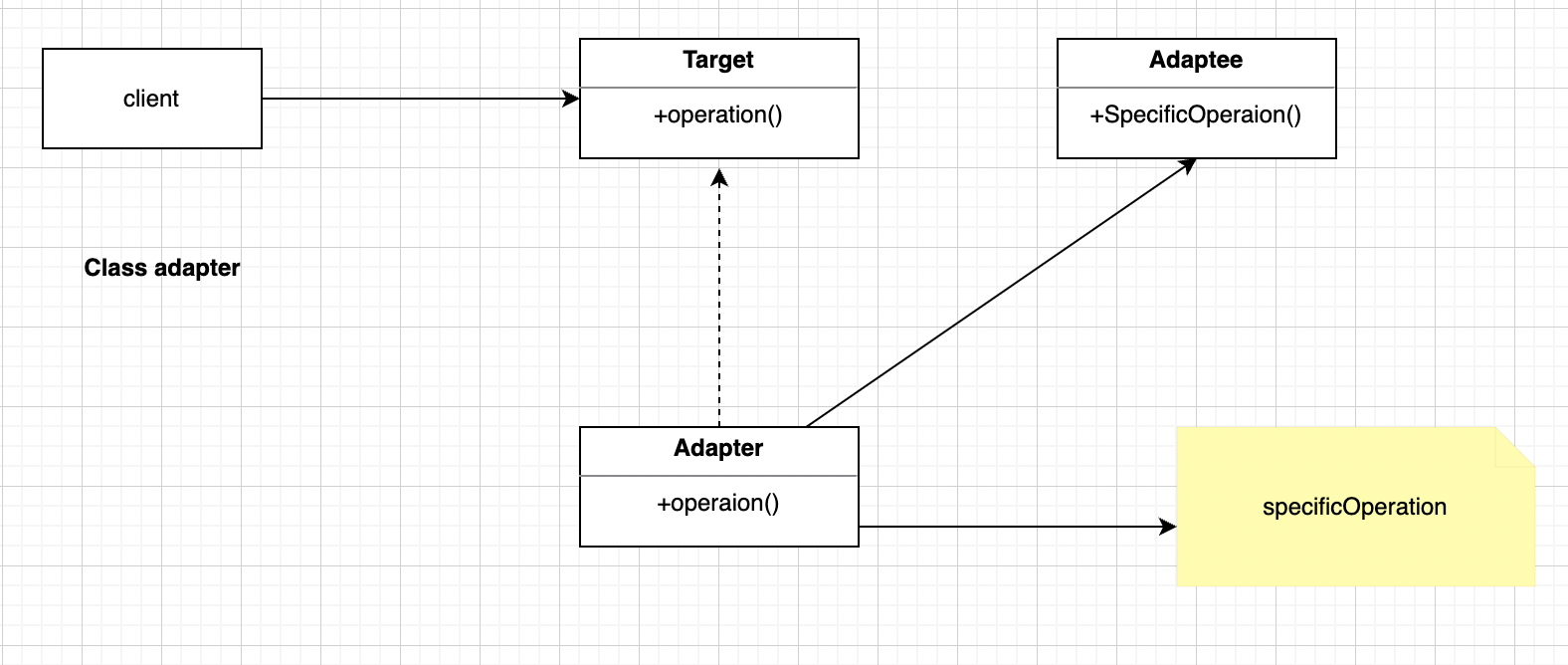
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| public class ClassAdapter { public static void main(String[] args) { Adapter adapter = new Adapter(); adapter.outPut5v(); } } class Adaptee{ public int outPut220v(){ return 220; } } interface Target{ int outPut5v(); }
class Adapter extends Adaptee implements Target{ @Override public int outPut5v() { int i = outPut220v(); System.out.println(String.format("原始电压 %d v -> 输出电压 %d v",i,5)); return 5; } }
|
应用场景:
1.当你希望使用某些现有类,但其接口与您的其他代码不兼容时,请使用适配器类。
2.当你希望重用几个现有的子类,这些子类缺少一些不能添加到超类中的公共功能时,请使用该模式。
优点:
1.符合单一职责原则、
2.符合开闭原则
适配器总结:
类适配器采用继承,对象适配器采用组合;
类适配器属于静态实现,对象适配器属于组合的动态实现,对象适配器需要多实例化一个对象。
总体来说,对象适配器用的比较多。
JDK & Spring源码中的应用
1 2 3 4 5
| JDK: java.util.Arrays#asList() java.util.Collections#list() Spring: org.springframework.context.event.GenericApplicationListenerAdapter
|